Extracting Pedestrian Networks: A Boundary-Based Approach
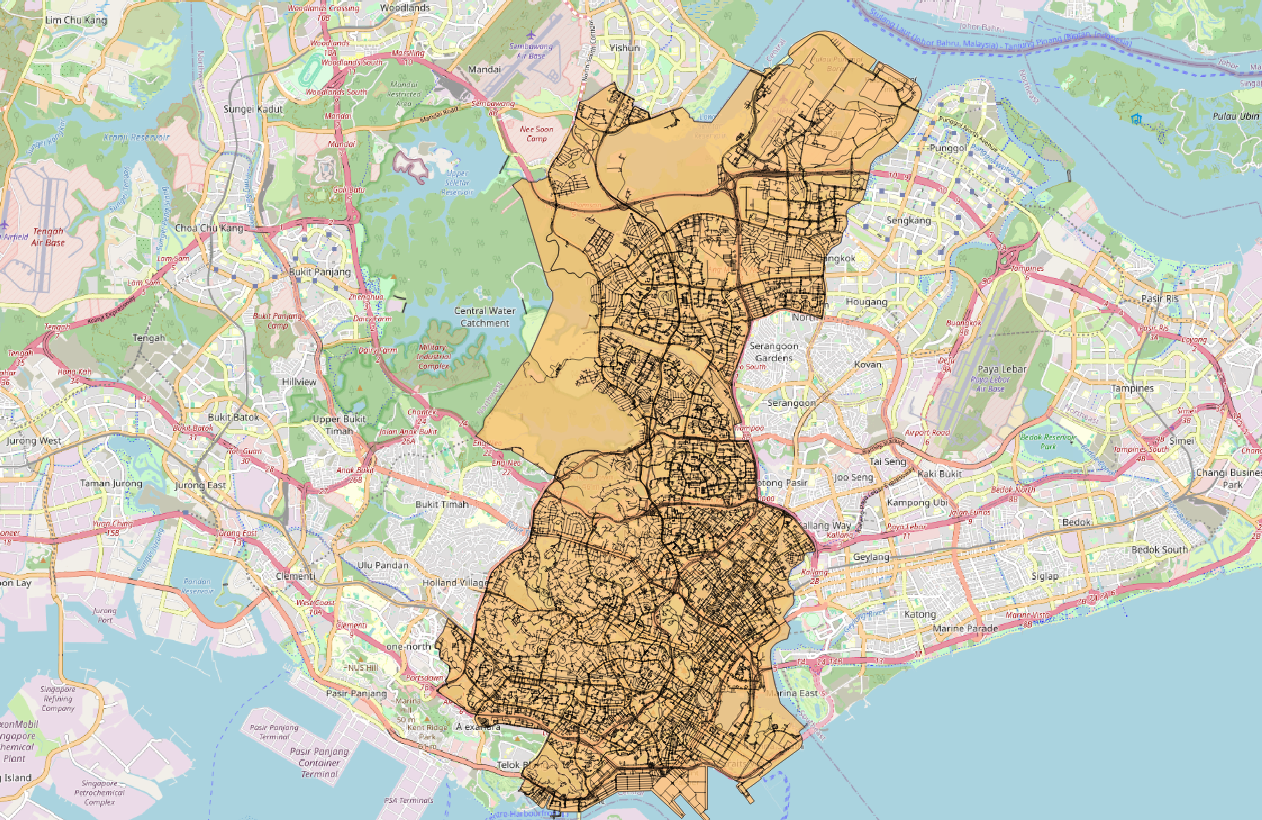
# Define the environment with the required libraries
#!pip install python3-rtree --quiet
!pip install rtree
!pip install osmnx
!pip install geopandas
!pip install geopy
!pip install networkx
# Import libraries
import osmnx as ox
import matplotlib.pyplot as plt
import geopandas as gpd
import networkx as nx
#from google.colab import files # Download the Saved Files in Google Colab
from shapely.geometry import Point, LineString
import os
After setting up our Python environment with the necessary libraries, the next crucial step is to define the geographical boundaries of the city we want to analyze. This information is pivotal for extracting the pedestrian network within the specified area.
Reading Boundary Files
For our project, we obtained boundary files in the .GPKG format for cities of interest, such as Jakarta, Manila, Phnom Penh, and Ho Chi Minh City. These files are crucial as they delineate the geographical extent of the city. Here are the links to the respective boundaries:
Reading the Boundary File
Now, let's dive into the code that reads and visualizes the boundary data. This function, read_boundary_data(url), takes a URL pointing to the boundary file in .GPKG format and returns a GeoDataFrame (gdf) containing the boundary geometries.
#### Read the boundary file
def read_boundary_data(url):
gdf = gpd.read_file(url)
#### Plot
# Create a basic plot
gdf.plot()
# Add title and labels
plt.title("Your boundary map")
plt.xlabel("Longitude")
plt.ylabel("Latitude")
# Show the plot
plt.show()
return gdf
url = "https://www.dropbox.com/scl/fi/xqlsgatcqw6o28pr544rv/boundary-polygon-land-lvl4.gpkg?rlkey=e3ztzm0zrx5ytc09bda41rft3&dl=1"
gdf = read_boundary_data(url)
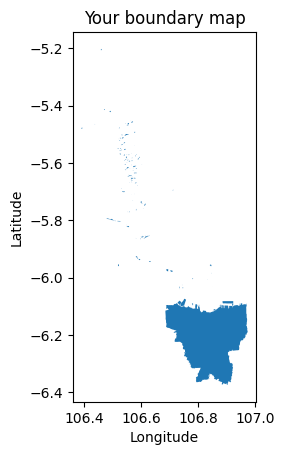
In this snippet, we use the geopandas library to read the boundary file specified by the URL. The resulting GeoDataFrame (gdf) contains the geometrical information of the city's boundary. This step is essential for defining the study area and extracting the pedestrian network within the specified city limits.